SharePlay
Overview
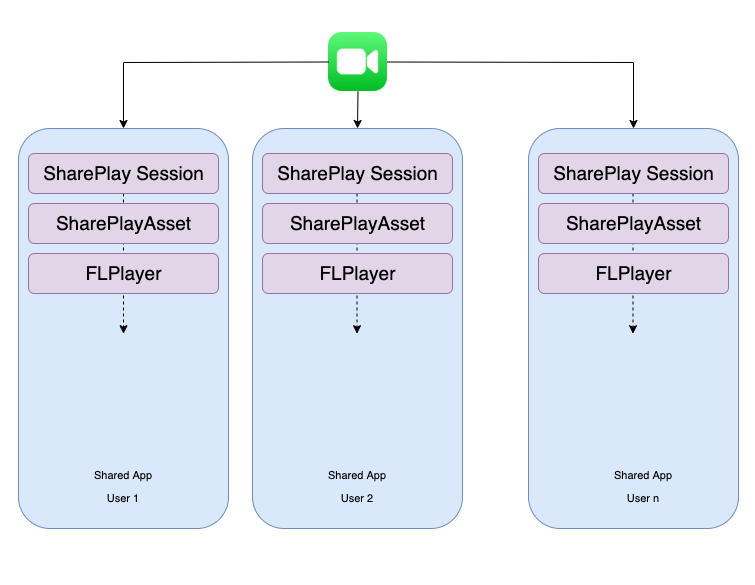
In a real world we do watch/listen movies or audios together with family or friends while having a real-time conversation, same kind of experience is now possible with virtual-world. SharePlay lets us watch/listen together while having a real-time conversation on FaceTime call. With smart volume, media audio is adjusted dynamically, so you can continue to chat while watching or listening.
Individual person watching the content can use the playback controls to play, pause, rewind, or fast-forward. (Settings like closed captioning and volume are controlled individually.)
You can use Picture in Picture to continue watching the video while using another app—order food, check your email, or jump into the Messages app and discuss the video by text when you want no interruptions to the sound of the movie or TV show.
Availability
- Application must be build with Xcode 13 or later and Swift 5.5 or later.
- Application must support iOS 15 or later.
Application Flow
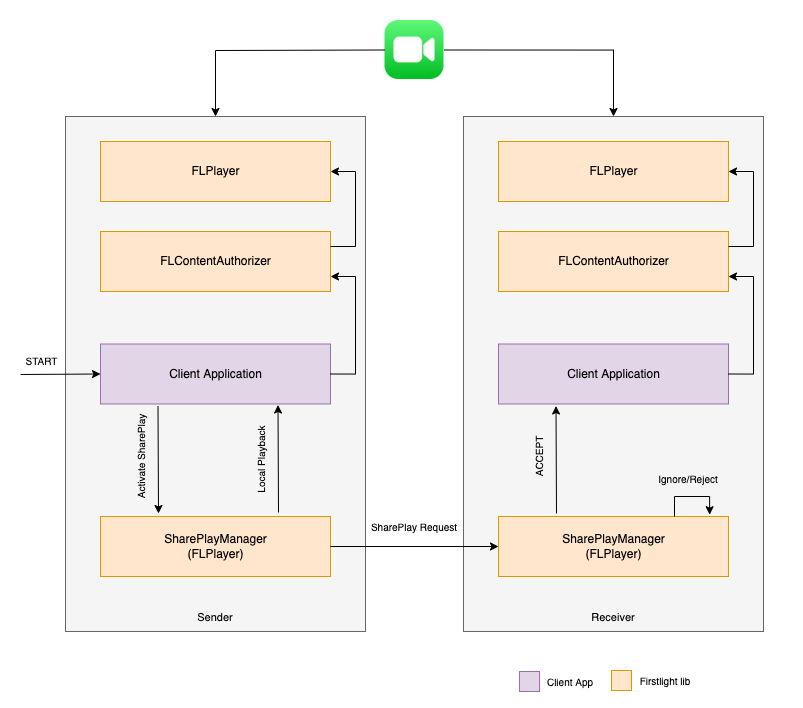
- While connected on a FaceTime call, sender will try to activate SharePlay using SharePlayAsset.
- SharePlayManager will now send request to everyone involved in a FaceTime call and trigger a delegate callback for local-playback.
- Receiver will accept the request followed by a delegate callback for remote-playback with SharePlayAsset.
- Both Sender and Receiver will now use received SharePlayAsset for ContentAuthorization followed by playback.
Usecases
- If one of the user don't have required streaming application which is used for SharePlay request, then other users will receive an app-store link to install that application,
fallbackURL
key must be used to pass the app-store url. - For the first-time, system will seek for an user consent from everyone involved in SharePlay.
- If a streaming application is not in back-ground, invitation will be received to launch the application.
- If a streaming application is already running or in background then content will start streaming directly in Picture-In-Picture mode in case of movies/shows.
- While on a SharePlay anyone can control and end the playback for everyone.
- Playback will be continued untill a Facetime call is in-progress meaning minimum two users on a FaceTime call can have no impact if rest others leave the FaceTime call.
SharePlay may not be in sync while playing SSAI integrated contents that has targeted ads.
Integration Guide
Add an Entitlement for Group Activities
<dict>
<key>com.apple.developer.group-session</key>
<true/>
</dict>
Please refere this apple document for more details.
Setup SharePlayManager delegate
SharePlayManager will hangle all the enablement required for SharePlay and trigger the delegate call-backs required for the application.
if #available(iOS 15.0, tvOS 15.0, *) {
FLPlayerFactory.sharePlayManager.delegate = self
}
Creating SharePlayAsset
Playback data must be constructed using SharePlayAsset
interface.
It requires:
PlatformAsset
object- Create a data dictinary of type
[String: String]
i.e.SharePlayMetadata
with all other details you need for a successful playback. Below are the keys used for displaying the SharePlay notification.- title: set this key with your desired media title
- previewImage: value of this key will be used for displaying the previw image
- fallbackURL: value of this key will be used to to handle the fallback behaviour for users who don’t have your app installed on their device.
let platformAsset = Quickplay.platformAsset(contentId: contentId, contentType: contentType,
catalogType: catalogType, mediaFormat: mediaFormat,
drm: drm, quality: .high, playbackMode: playbackMode,
startTime: ISO8601DateFormatter().string(from: startTime),
endTime: ISO8601DateFormatter().string(from: endTime))
var playbackData: SharePlayMetadata = ["title": catalogItem?.title ?? "Sample Title",
"previewImage": "https://your-preview-image-url-string",
"fallbackURL": "https://your-fallback-url-string"]
if let initialPlaybackValue = self.playbackStartTimeTextField.text {
playbackData["initialPlaybackValue"] = initialPlaybackValue
}
let shareplayAsset = FLPlayerFactory.assetForSharePlay(asset: platformAsset, data: playbackData)
Sharing activity data with SharePlayManager
On a play button click, share this activity data to enable the SharePlay.
if #available(iOS 15.0, tvOS 15.0, *), FLPlayerFactory.sharePlayManager.canSharePlay() {
let shareplayAsset = FLPlayerFactory.assetForSharePlay(asset: platformAsset, data: playbackData)
FLPlayerFactory.sharePlayManager.sharePlay(with: shareplayAsset)
}
Adopting SharePlayDelegate
func didReceiveAssetForSharePlay(asset: SharePlayAsset?) {
// Make sure authorizer is initialised
// Create the objects required for playback from the received `SharePlayAsset` object
guard let mediaAsset = asset else { return }
let platformAsset = mediaAsset.platformAsset
let initialPlaybackValue = mediaAsset["initialPlaybackValue"]
DispatchQueue.main.asyncAfter(deadline: .now() + 0.1) {
// Authorization and present the player with the received data
}
}
func didEndSharePlaySession() {
// You may like to close the active/on-screen player
}
func didFailWith(error: FLError) {
// Error handling
}
func didUpdateSharePlay(eligibility _: Bool) {
// You may like to update your UI as per SharePlay Eligibility
}
Prepare for Coordinated Playback
To have a coordinated playback and syncup between all the users, below API must be called using Player instance.
if #available(iOS 15.0, tvOS 15.0, *), FLPlayerFactory.sharePlayManager.isConnected() {
FLPlayerFactory.sharePlayManager.coordinate(with: player)
}
For further reading refere SharePlay and Group Activities section of apple document.